[unity] 유니티 쉐이더 공부 1
2021. 9. 4. 01:51
지난 번에 공부했던 <유니티 쉐이더 스타트업>의 내용을 정리해두지 않으면 올해 안에 완벽하게 까먹을 것 같아서
처음부터 기록+복습해보고자 한다.
학교에서 GLSL은 조금(정말 조금) 배웠으니 이 책에서 surface shader를 배운 후에는 GLSL을 더 공부해 보는 것이 좋을 것 같다
Part 2
- 렌더링 파이프 라인
1. 오브젝트 데이터 받아오기
2. vertex shader
3. rasterizer
4. pixel shader/fragment shader
(학교에서 배운 내용!! 뻥 안치고 한 학기동안 파이프라인 100번은 들음)
Part 4
3d object로 sphere 만들기
surface shader이랑 material 만들기
sphere에 material을 적용한 모습(material에 shader 적용되어있음)
_Name()에서의 언더바는 외부에서 입력받았다는 것을 표시하기 위해 자주 쓰임
properties 만들어보기
Shader "NewShader"
{
Properties
{
_Brightness("change Brightness!",Range(0,1))=0.5
_TextFloat("test float",Float)=0.3
_TextVector("test vector",Vector)=(1,1,1,1)
_TestColor ("test Color", Color) = (1,1,1,1)
_TestTexture("test texture",2D)="white"{}
_Color ("Color", Color) = (1,1,1,1)
_MainTex ("Albedo (RGB)", 2D) = "white" {}
_Glossiness ("Smoothness", Range(0,1)) = 0.5
_Metallic ("Metallic", Range(0,1)) = 0.0
}
void surf (Input IN, inout SurfaceOutputStandard o)
{
fixed4 c = tex2D (_MainTex, IN.uv_MainTex) * _Color;
o.Albedo = float3(1,0,0);
o.Alpha = c.a;
}
o.Albedo를 (1,0,0)으로 바꿈
Albedo를 Emission으로 바꿈
Emission은 조명연산을 받지 않음
void surf (Input IN, inout SurfaceOutputStandard o)
{
fixed4 c = tex2D (_MainTex, IN.uv_MainTex) * _Color;
o.Emission = float3(1,0,0);
o.Alpha = c.a;
}
변수 사용해보기
void surf (Input IN, inout SurfaceOutputStandard o)
{
float4 test=float4(1,0,0,0);
fixed4 c = tex2D (_MainTex, IN.uv_MainTex) * _Color;
o.Albedo = test.rgb;
o.Alpha = c.a;
}
r과 g 바꿔보기
void surf (Input IN, inout SurfaceOutputStandard o)
{
float4 test=float4(1,0,0,0);
fixed4 c = tex2D (_MainTex, IN.uv_MainTex) * _Color;
o.Albedo = test.grb;
o.Alpha = c.a;
}
void surf (Input IN, inout SurfaceOutputStandard o)
{
float r=1;
float2 gg=float2(0.5,0);
float3 bbb=float3(1,0,1);
fixed4 c = tex2D (_MainTex, IN.uv_MainTex) * _Color;
o.Albedo =float3(r,0,0);
o.Alpha = c.a;
}
당연히...r=1으로 할당했으니까
void surf (Input IN, inout SurfaceOutputStandard o)
{
float r=1;
float2 gg=float2(0.5,0);
float3 bbb=float3(1,0,1);
fixed4 c = tex2D (_MainTex, IN.uv_MainTex) * _Color;
o.Albedo =float3(0,gg);
o.Alpha = c.a;
}
Shader "Custom/shader5"
{
Properties
{
_Color ("Color", Color) = (1,1,1,1)
_MainTex ("Albedo (RGB)", 2D) = "white" {}
_Glossiness ("Smoothness", Range(0,1)) = 0.5
_Metallic ("Metallic", Range(0,1)) = 0.0
_TestColor("testcolor",Color)=(1,1,1,1)
}
SubShader
{
Tags { "RenderType"="Opaque" }
LOD 200
CGPROGRAM
// Physically based Standard lighting model, and enable shadows on all light types
#pragma surface surf Standard fullforwardshadows
// Use shader model 3.0 target, to get nicer looking lighting
#pragma target 3.0
sampler2D _MainTex;
struct Input
{
float2 uv_MainTex;
};
half _Glossiness;
half _Metallic;
fixed4 _Color;
fixed4 _TestColor;
void surf (Input IN, inout SurfaceOutputStandard o)
{
fixed4 c = tex2D (_MainTex, IN.uv_MainTex) * _Color;
o.Albedo = _TestColor.rgb;
o.Metallic = _Metallic;
o.Smoothness = _Glossiness;
o.Alpha = c.a;
}
ENDCG
}
FallBack "Diffuse"
}
Shader "Custom/shader6"
{
Properties
{
_Color ("Color", Color) = (1,1,1,1)
_MainTex ("Albedo (RGB)", 2D) = "white" {}
_Glossiness ("Smoothness", Range(0,1)) = 0.5
_Metallic ("Metallic", Range(0,1)) = 0.0
_Red("red",Range(0,1))=0
_Green("green",Range(0,1))=0
_Blue("blue",Range(0,1))=0
}
SubShader
{
Tags { "RenderType"="Opaque" }
LOD 200
CGPROGRAM
// Physically based Standard lighting model, and enable shadows on all light types
#pragma surface surf Standard fullforwardshadows
// Use shader model 3.0 target, to get nicer looking lighting
#pragma target 3.0
sampler2D _MainTex;
struct Input
{
float2 uv_MainTex;
};
half _Glossiness;
half _Metallic;
fixed4 _Color;
float _Red;
float _Green;
float _Blue;
void surf (Input IN, inout SurfaceOutputStandard o)
{
fixed4 c = tex2D (_MainTex, IN.uv_MainTex) * _Color;
o.Albedo = float3(_Red,_Green,_Blue);
o.Alpha = c.a;
}
ENDCG
}
FallBack "Diffuse"
}
Shader "Custom/shader7"
{
Properties
{
_Color ("Color", Color) = (1,1,1,1)
_MainTex ("Albedo (RGB)", 2D) = "white" {}
_Glossiness ("Smoothness", Range(0,1)) = 0.5
_Metallic ("Metallic", Range(0,1)) = 0.0
_Red("red",Range(0,1))=0
_Green("green",Range(0,1))=0
_Blue("blue",Range(0,1))=0
_BrightDark("brightness & darkness",Range(0,1))=0.5
}
SubShader
{
Tags { "RenderType"="Opaque" }
LOD 200
CGPROGRAM
// Physically based Standard lighting model, and enable shadows on all light types
#pragma surface surf Standard fullforwardshadows
// Use shader model 3.0 target, to get nicer looking lighting
#pragma target 3.0
sampler2D _MainTex;
struct Input
{
float2 uv_MainTex;
};
half _Glossiness;
half _Metallic;
fixed4 _Color;
float _Red;
float _Green;
float _Blue;
float _BrightDark;
void surf (Input IN, inout SurfaceOutputStandard o)
{
fixed4 c = tex2D (_MainTex, IN.uv_MainTex) * _Color;
o.Albedo = float3(_Red,_Green,_Blue)+_BrightDark;
o.Alpha = c.a;
}
ENDCG
}
FallBack "Diffuse"
}
_BrightDark("brightness & darkness",Range(-1,1))=0.5
어두워질 수 있도록 수정
좀 더 깔끔하게 지우기
Shader "Custom/shader8"
{
Properties
{
_Red("red",Range(0,1))=0
_Green("green",Range(0,1))=0
_Blue("blue",Range(0,1))=0
_BrightDark("brightness & darkness",Range(0,1))=0.5
}
SubShader
{
Tags { "RenderType"="Opaque" }
LOD 200
CGPROGRAM
// Physically based Standard lighting model, and enable shadows on all light types
#pragma surface surf Standard fullforwardshadows
// Use shader model 3.0 target, to get nicer looking lighting
#pragma target 3.0
struct Input
{
float4 color:COLOR;
};
float _Red;
float _Green;
float _Blue;
float _BrightDark;
void surf (Input IN, inout SurfaceOutputStandard o)
{
o.Albedo = float3(_Red,_Green,_Blue)+_BrightDark;
o.Alpha =1;
}
ENDCG
}
FallBack "Diffuse"
}
깔끔!
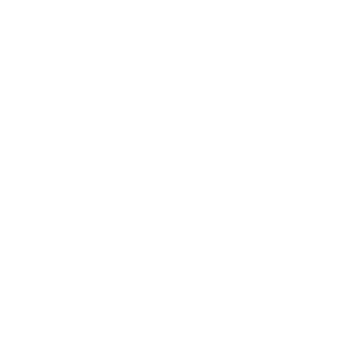
Shader "Custom/shader8"
{
Properties
{
_Red("red",Range(0,1))=0
_Green("green",Range(0,1))=0
_Blue("blue",Range(0,1))=0
_BrightDark("brightness & darkness",Range(0,1))=0.5
}
SubShader
{
Tags { "RenderType"="Opaque" }
CGPROGRAM
#pragma surface surf Standard fullforwardshadows
struct Input
{
float4 color:COLOR;
};
float _Red;
float _Green;
float _Blue;
float _BrightDark;
void surf (Input IN, inout SurfaceOutputStandard o)
{
o.Albedo = float3(_Red,_Green,_Blue)+_BrightDark;
o.Alpha =1;
}
ENDCG
}
FallBack "Diffuse"
}
더 깔끔!
'컴퓨터 > graphics' 카테고리의 다른 글
[unity] 유니티 URP 셰이더 1 (0) | 2022.08.06 |
---|---|
[unity] 유니티 쉐이더 공부 4 (0) | 2022.02.22 |
[unity] 유니티 쉐이더 공부 3 (0) | 2021.09.08 |
[unity] 유니티 쉐이더 공부 2 (0) | 2021.09.05 |